Note
Click here to download the full example code
Greenstone.¶
# Importing gempy
import gempy as gp
# Aux imports
import numpy as np
import matplotlib.pyplot as plt
import os
print(gp.__version__)
Out:
2.2.8
geo_model = gp.create_model('Greenstone')
data_path = 'https://raw.githubusercontent.com/cgre-aachen/gempy_data/master/'
# Importing the data from csv files and settign extent and resolution
geo_model = gp.init_data(geo_model, [696000, 747000, 6863000, 6930000, -20000, 200], [50, 50, 50],
path_o=data_path + "/data/input_data/tut_SandStone/SandStone_Foliations.csv",
path_i=data_path + "/data/input_data/tut_SandStone/SandStone_Points.csv")
Out:
Active grids: ['regular']
gp.plot_2d(geo_model, direction=['z'])
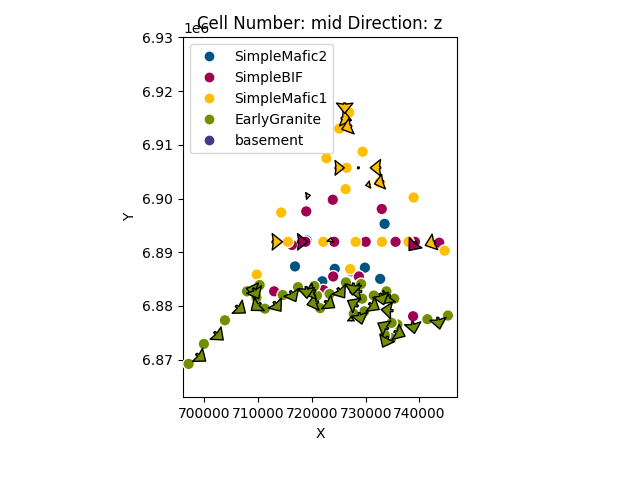
Out:
<gempy.plot.visualization_2d.Plot2D object at 0x7fcc6b85fb80>
gp.map_stack_to_surfaces(geo_model, {"EarlyGranite_Series": 'EarlyGranite',
"BIF_Series": ('SimpleMafic2', 'SimpleBIF'),
"SimpleMafic_Series": 'SimpleMafic1', 'Basement': 'basement'})
geo_model.add_surface_values([2.61, 2.92, 3.1, 2.92, 2.61])
gp.set_interpolator(geo_model,
compile_theano=True,
theano_optimizer='fast_compile',
verbose=[])
Out:
Setting kriging parameters to their default values.
Compiling theano function...
Level of Optimization: fast_compile
Device: cpu
Precision: float64
Number of faults: 0
Compilation Done!
Kriging values:
values
range 86591.22
$C_o$ 178524761.9
drift equations [3, 3, 3, 3]
<gempy.core.interpolator.InterpolatorModel object at 0x7fcc2a5a6130>
gp.compute_model(geo_model, set_solutions=True)
Out:
Lithology ids
[5. 5. 5. ... 5. 5. 5.]
gp.plot_2d(geo_model, cell_number=[-1], direction=['z'], show_data=False)
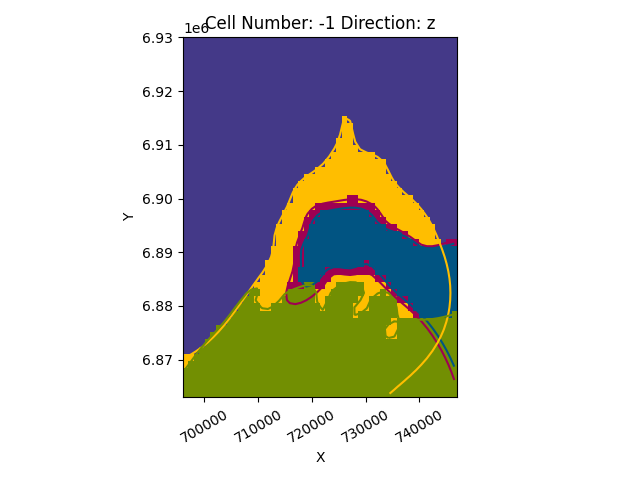
Out:
<gempy.plot.visualization_2d.Plot2D object at 0x7fcc6b69bf10>
gp.plot_2d(geo_model, cell_number=[25], direction='x')
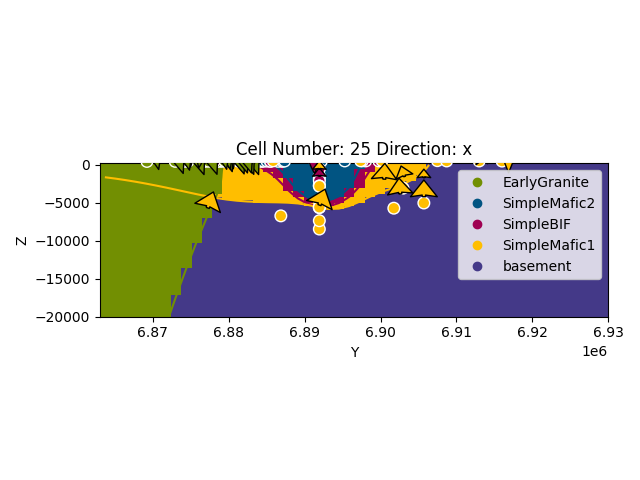
Out:
<gempy.plot.visualization_2d.Plot2D object at 0x7fcc3283c5b0>
Out:
array([[2.61, 2.61, 2.61, ..., 2.61, 2.61, 2.61]])
p2d = gp.plot_2d(geo_model, cell_number=[25], block=geo_model.solutions.values_matrix,
direction=['y'], show_data=True,
kwargs_regular_grid={'cmap': 'viridis', 'norm':None})
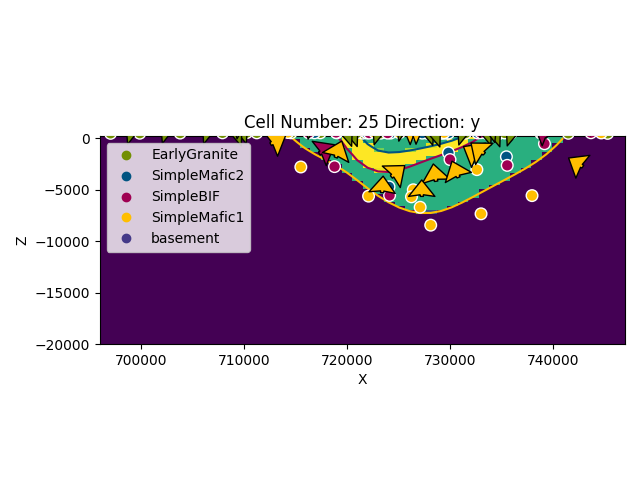
sphinx_gallery_thumbnail_number = 5
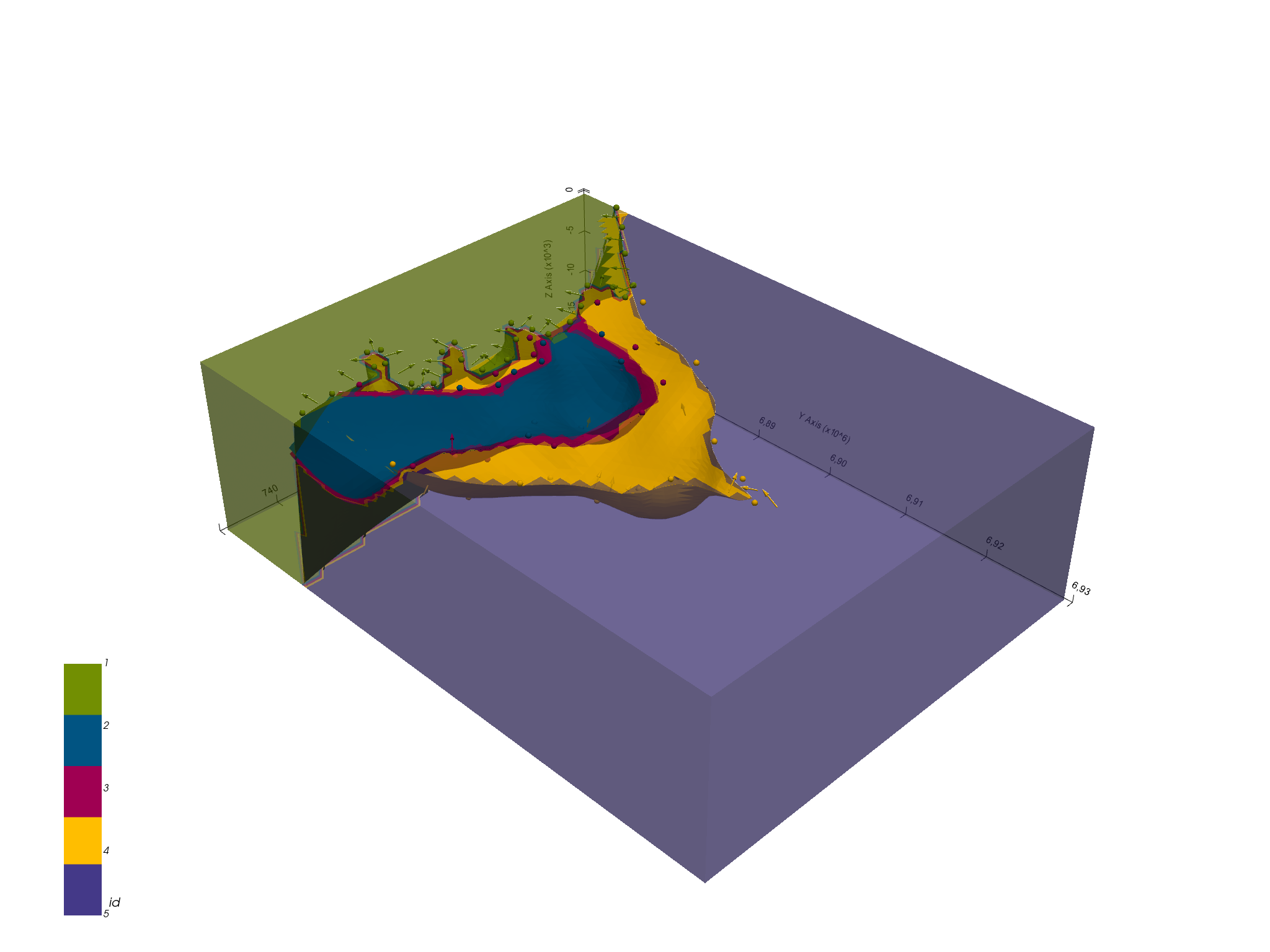
Out:
<gempy.plot.vista.GemPyToVista object at 0x7fcc2a5cd7c0>
np.save('greenstone_ver', geo_model.solutions.vertices)
np.save('greenstone_edges', geo_model.solutions.edges)